JTree is a Swing class used display a tree like structure such as the one shown below.
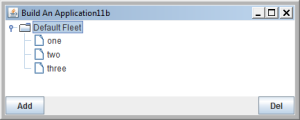
Now unlike some of the more basic swing objects JTree is designed to display a tree structure, another object is used to hold the data which determines what JTree displays.
This other object is the TreeModel. Now that is not to say that you have to use a model to get JTree to display something, you don’t and we can take a look at that in a while.
JTree displays information presented to it in the form of TreeNodes. So in the image above “Default Fleet”, “one”, “two” and “three” are all presented to the JTree object as TreeNodes.
In fact, if we wanted to create a JTree we could simply call any one of a number of constructors and it would give us a JTree. To demonstrate the point we could do this:
// create an array of strings final String[] toppings = {"Cheese", "Pepperoni", "Black Olives"}; public class JTreePanelOne { private JPanel jpanel = new JPanel(); private JTree treeOne; private String []options; public JTreePanelOne(String vals[]) { super(); options = vals; treeOne = new JTree(options); jpanel.add(treeOne); } public JPanel getJpanel() { return jpanel; } }
And this would give us a JTree that looked like this:

Now as we can see this displays a simply JTree with no hierarchy.
The other challenge with this approach is it is difficult to change, if you want to add or remove an item JTree does not provide an obvious way to achieve this.
That is not to say you could not. JTree creates a TreeModel if it is not passed one and this can be accessed by calling the JTree.getModel. From that we can call the getRoot() and getChildCount(root), iterate through the model using getChild(root, index) which would return an Object for each child.
Obviously this is straight forward for our simple tree structure but it gets more complicated when nodes have children which in turn also have children.
The point here is that we can get a TreeModel from JTree but this is not perhaps the easiest way to handle things. In the next posting in this series we will look at implementing a JTree with a hierarchy.